1. Abstract
This thesis presents the design and implementation of a GPRS-based monitoring system for industrial environments. The system collects real-time data from various sensors (temperature, humidity, etc.), transmits the data through a GPRS module, and provides a web interface for remote monitoring. The objective is to enhance operational efficiency by offering a cost-effective and reliable solution for industrial monitoring. Testing shows the system’s effectiveness in maintaining real-time data accuracy over long distances.
2. Introduction
- Problem Statement: In many industries, monitoring critical parameters like temperature, pressure, and humidity is essential. Traditional monitoring systems require manual checking, which is time-consuming and prone to error. A GPRS-based system allows for real-time, remote monitoring using cellular networks, enabling prompt responses to critical situations.
- Objective: Develop an efficient and cost-effective system that can monitor industrial parameters remotely and in real time using GPRS technology.
- Scope: The project covers designing a system that gathers data from sensors, processes it through a microcontroller, and sends it to a remote server via GPRS. The scope also includes designing a web interface for visualizing the data.
- Need for GPRS-Based Industrial Monitoring System
- Remote Monitoring of Industrial Processes:
Industrial facilities often span vast areas or are located in remote, hard-to-reach places. There is a growing need for a solution that allows operators to monitor processes, machinery, and environmental conditions remotely without requiring constant on-site presence. A GPRS-based monitoring system enables real-time tracking from any location, reducing the need for physical inspections. - Minimizing Downtime and Increasing Efficiency:
In industries where downtime can lead to significant financial losses, having a real-time monitoring system is critical. The GPRS-based system allows for instant alerts in case of abnormal conditions or equipment failure, enabling immediate intervention to prevent prolonged downtime. This ensures that operations remain smooth and efficient. - Cost-Effective Data Transmission:
Traditional methods of long-range communication, such as satellite or wired connections, can be expensive and require significant infrastructure. GPRS, on the other hand, offers a cost-effective solution, as it leverages existing cellular networks for data transmission. This minimizes setup and operational costs, making it suitable for industries with limited budgets. - Scalable and Flexible Monitoring Solution:
Many industries require a flexible monitoring solution that can be scaled as operations grow. A GPRS-based system allows easy integration of additional sensors and devices without the need for major infrastructure changes. This scalability makes it an ideal choice for evolving industrial environments. - Real-Time Data for Proactive Maintenance:
The need for predictive and condition-based maintenance is increasing across industries. Real-time data collected by the GPRS system helps detect early signs of equipment wear or failure, allowing operators to perform maintenance before a major breakdown occurs. This reduces maintenance costs and improves operational safety. - Enhancing Safety in Hazardous Environments:
Certain industries, such as chemical processing, oil and gas, or mining, involve dangerous working conditions. Remote monitoring using GPRS reduces the need for personnel to enter hazardous environments to perform manual inspections, enhancing worker safety and minimizing risk. - Global Accessibility and Wide Network Coverage:
GPRS offers the advantage of being globally accessible due to the widespread availability of cellular networks. This makes it ideal for monitoring industrial sites that are located in rural or geographically dispersed areas where other communication methods might not be feasible. - Improved Decision-Making through Data Analytics:
Continuous real-time data collection enables industries to perform trend analysis, predict potential issues, and optimize operational strategies. GPRS-based systems facilitate data-driven decision-making, improving overall efficiency and resource management
3. Literature Review
- Existing Solutions: A review of existing industrial monitoring technologies, including SCADA systems, GSM-based systems, and wireless sensor networks (WSN). These systems have limitations such as high cost or limited range, which GPRS technology overcomes by offering a broad coverage area and cost-effectiveness.
- GPRS Technology: GPRS (General Packet Radio Service) allows for data transmission over cellular networks. It is a key enabler for Internet of Things (IoT) systems due to its wide availability and lower cost compared to other wireless communication technologies like 4G or Wi-Fi.
- Gap Analysis: Most current systems are expensive or lack the capability to cover large geographic areas. GPRS-based monitoring offers a solution by leveraging existing cellular networks for long-distance data transmission without significant infrastructure costs.
4. System Design
System Architecture
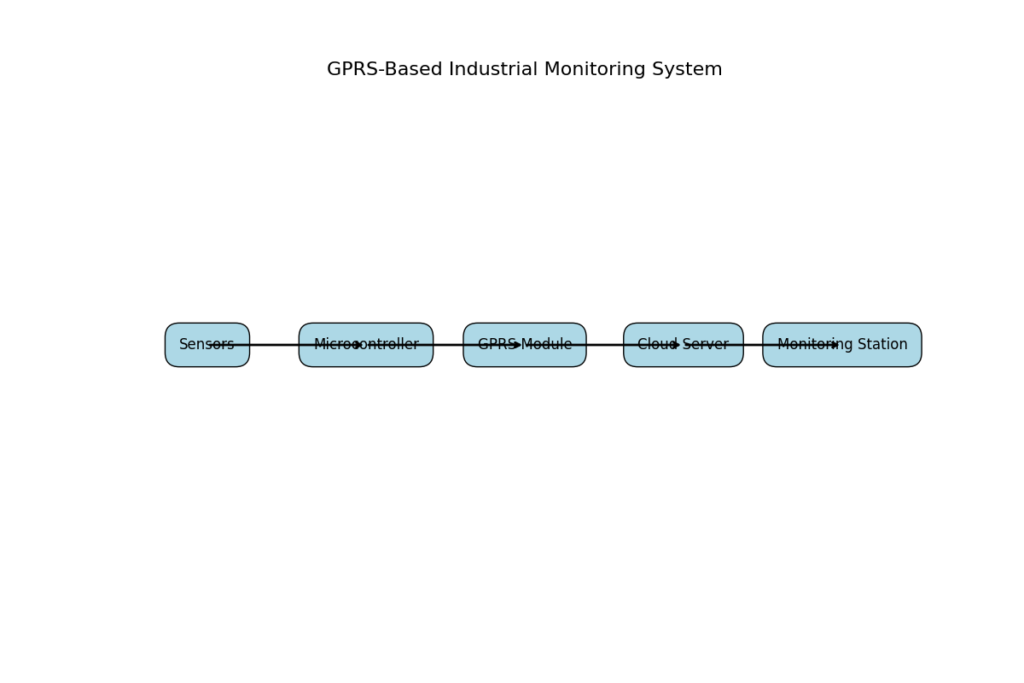
The system consists of the following main components:
- Sensors: Collect data such as temperature, humidity, and pressure. These sensors are connected to a microcontroller.
- Microcontroller: Arduino or ESP8266 microcontroller is used to process the data from sensors and communicate with the GPRS module.
- GPRS Module: A SIM900 or SIM800 module connects to the microcontroller and transmits the data over a cellular network to a remote server.
- Server: A web server receives the data sent from the microcontroller via GPRS, stores it in a database, and displays it on a web interface.
- User Interface: A web-based dashboard that shows real-time data from the sensors. The interface allows users to monitor industrial parameters remotely.
Hardware Components
- Microcontroller (Arduino Uno): The brain of the system, responsible for gathering sensor data and controlling the GPRS module.
- GPRS Module (SIM900): Responsible for transmitting data over the mobile network.
- Sensors: Depending on the parameters to monitor, you may use:
- Temperature sensor (LM35)
- Humidity sensor (DHT11)
- Pressure sensor (BMP180)
- Power Supply: Powering the microcontroller and GPRS module. For portable or off-grid applications, a battery pack may be used.
- Miscellaneous Components: Resistors, capacitors, connectors, and jumper wires for assembling the circuit.
5. Implementation
Hardware Setup
- Sensor Connections:
- Temperature Sensor (LM35): Connect the sensor to one of the analog pins of the microcontroller (e.g., A0 for Arduino). The other pins go to power (Vcc) and ground (GND).
- Humidity Sensor (DHT11): Connect it to another GPIO pin (e.g., D3) for digital data reading.
- GPRS Module Connections:
- Connect TX pin of SIM900 to the RX pin of the Arduino.
- Connect RX pin of SIM900 to the TX pin of the Arduino.
- Power the GPRS module using the 5V pin from the Arduino.
- Insert a working SIM card into the GPRS module.
Software Implementation
Arduino Code:
This code will collect data from sensors and send it to a web server via the GPRS module.
#include <SoftwareSerial.h>
#include <DHT.h>
#define DHTPIN 2
#define DHTTYPE DHT11
DHT dht(DHTPIN, DHTTYPE);
SoftwareSerial sim900(7, 8); // RX, TX pins for SIM900
String server = "http://example.com/upload.php"; // Server URL
void setup() {
Serial.begin(9600);
sim900.begin(9600);
dht.begin();
// Initialize GPRS
sim900.println("AT"); // Test communication
delay(1000);
sim900.println("AT+CSTT=\"your_apn\""); // Set APN
delay(1000);
sim900.println("AT+CIICR"); // Bring up wireless connection
delay(3000);
sim900.println("AT+CIFSR"); // Get IP address
}
void loop() {
float h = dht.readHumidity(); // Read humidity
float t = dht.readTemperature(); // Read temperature
// Send data via GPRS to server
String data = "temp=" + String(t) + "&humidity=" + String(h);
sendData(data);
delay(60000); // Wait for 1 minute before sending data again
}
void sendData(String data) {
sim900.println("AT+HTTPINIT"); // Initialize HTTP service
delay(1000);
sim900.println("AT+HTTPPARA=\"URL\",\"" + server + "?" + data + "\""); // Set server URL with data
delay(1000);
sim900.println("AT+HTTPACTION=0"); // Send HTTP GET request
delay(3000);
sim900.println("AT+HTTPTERM"); // Terminate HTTP service
delay(1000);
}
Explanation of Code:
- The DHT11 sensor reads temperature and humidity.
- The SIM900 module sends the data to a server using an HTTP GET request.
AT commands
are used to control the GPRS module for initializing, setting APN, and sending data.
Server-Side Code:
A simple PHP script can be used on the server to receive and store the data.
<?php
$temperature = $_GET['temp'];
$humidity = $_GET['humidity'];
// Connect to MySQL database
$conn = new mysqli('localhost', 'username', 'password', 'database');
// Insert data into database
$sql = "INSERT INTO sensor_data (temperature, humidity) VALUES ('$temperature', '$humidity')";
$conn->query($sql);
echo "Data saved successfully";
?>
User Interface:
You can use HTML, CSS, and JavaScript for a basic web interface that fetches and displays the data from the server.
6. Testing and Results
- Testing Environment: The system was tested in a simulated industrial environment. Sensors were placed near machines generating heat and humidity.
- Test Cases:
- Successfully transmitting sensor data over long distances.
- Monitoring real-time data on the web dashboard.
- GPRS reliability in various locations.
- Results: The system successfully transmitted data over a wide range using GPRS. Real-time monitoring was effective, with minimal latency.
7. Discussion
- Challenges:
- Network instability was observed in areas with weak GPRS signals.
- Power consumption of the GPRS module required an external power supply for extended operations.
- Performance: The system performed well in terms of accuracy and reliability. Data transmission speeds were acceptable for real-time monitoring.
8. Conclusion and Future Work
- Conclusion: The GPRS-based monitoring system proved to be a cost-effective solution for real-time industrial monitoring. It allows for remote monitoring over long distances without the need for a dedicated wireless infrastructure.
- Future Work: Future improvements can include using LTE or 4G modules for faster data transmission, integrating machine learning algorithms for predictive maintenance, and adding more sensors for comprehensive monitoring.
Applications and Advantages of the GPRS-Based Industrial Monitoring System
Applications
- Remote Industrial Monitoring:
- Used in industries such as manufacturing, oil & gas, and power plants to monitor environmental conditions like temperature, humidity, and pressure in real-time.
- Reduces the need for manual inspections, ensuring consistent monitoring of critical parameters even in hazardous or hard-to-reach areas.
- Smart Grid Monitoring:
- In smart grids, the system can be used to monitor transformers, substations, and distribution lines, providing real-time data to optimize performance and detect faults quickly.
- The GPRS-based system can send alerts when abnormal conditions occur, facilitating proactive maintenance.
- Agriculture and Environmental Monitoring:
- Can be employed in agriculture to monitor soil moisture, temperature, and humidity in greenhouses or large-scale farms.
- Environmental agencies can use it to monitor air and water quality over a vast geographical area, utilizing the wide coverage of GPRS technology.
- Building Management Systems:
- The system can monitor parameters like temperature, humidity, and CO2 levels in large buildings or campuses, enabling centralized control for energy efficiency and safety.
- Oil & Gas Pipelines:
- Useful in monitoring pipeline pressure, temperature, and flow rates, enabling early detection of leaks or other anomalies.
- GPRS coverage helps monitor pipelines over long distances in remote locations.
- Water Treatment and Distribution Plants:
- Used to monitor water levels, flow, and pressure in treatment plants and distribution networks, ensuring operational efficiency and the early detection of problems.
- Renewable Energy Systems:
- Can monitor wind turbines, solar panels, or other renewable energy systems for performance and fault detection, helping maintain optimal operational efficiency.
Advantages
- Cost-Effective Solution:
- Minimal Infrastructure: Since GPRS utilizes existing cellular networks, there is no need to invest in proprietary communication infrastructure like Wi-Fi or satellite, making it a cost-effective solution for long-range monitoring.
- Low Data Costs: GPRS is ideal for applications that require small amounts of data transmission at low frequency, reducing overall operational costs.
- Wide Geographical Coverage:
- Global Connectivity: GPRS is supported by almost all mobile networks, making it suitable for industries located in remote or geographically dispersed locations, where other forms of connectivity may not be available.
- Rural Areas: Ideal for industries and applications operating in rural areas or across large distances where Wi-Fi or Ethernet connections may not reach.
- Real-Time Data Monitoring:
- Immediate Alerts: The system provides real-time data, enabling immediate decision-making, especially in critical industrial environments where rapid responses are essential to prevent system failures or accidents.
- Remote Access: Data can be accessed from anywhere using a web interface or mobile app, allowing for real-time monitoring even when operators are off-site.
- Scalability:
- Flexible Design: The system can easily scale to include more sensors or monitor additional parameters without major infrastructure changes.
- Multi-Site Monitoring: Multiple industrial sites can be monitored from a centralized location, reducing the need for on-site personnel and allowing for easier management.
- Ease of Implementation:
- Simple Setup: The use of a GPRS module and a microcontroller (such as Arduino) makes the system easy to design, implement, and modify. Components like SIM900 modules are widely available and require minimal configuration.
- Compatibility with Existing Systems: The system can be integrated with existing monitoring solutions, adding GPRS connectivity for remote access without overhauling the entire infrastructure.
- Reliability and Stability:
- Dependable Network: GPRS has been proven reliable over the years, especially for industrial applications where only small amounts of data need to be sent intermittently.
- Backup Communication: GPRS can act as a backup communication network in case other methods like Wi-Fi or LAN go down, ensuring continued monitoring without interruptions.
- Data Logging and Analytics:
- Historical Data: The system can store data on a server, allowing operators to analyze historical data and identify trends. This is useful for predictive maintenance, as recurring patterns can indicate impending system failures.
- Better Decision-Making: Continuous monitoring and historical data allow companies to make informed decisions about operational improvements, resource allocation, and system upgrades.
- Low Power Consumption:
- Energy-Efficient: Compared to 3G or 4G LTE, GPRS consumes relatively less power, making it suitable for battery-operated or off-grid applications where power resources are limited.
- Proactive Maintenance:
- Condition-Based Maintenance: By providing real-time data and early alerts, the system enables condition-based maintenance, reducing the frequency of unexpected breakdowns and minimizing downtime.
- Reduced Maintenance Costs: Remote monitoring helps avoid costly, time-consuming manual inspections, particularly in hazardous or difficult-to-access areas.
- Improved Safety:
- Hazardous Environments: The ability to remotely monitor conditions in hazardous industrial environments (e.g., chemical plants or oil refineries) reduces the need for human presence, enhancing worker safety.
- Preventive Alerts: The system can issue early warnings in case of dangerous conditions like gas leaks, overheating, or machinery malfunctions, enabling swift preventive measures.