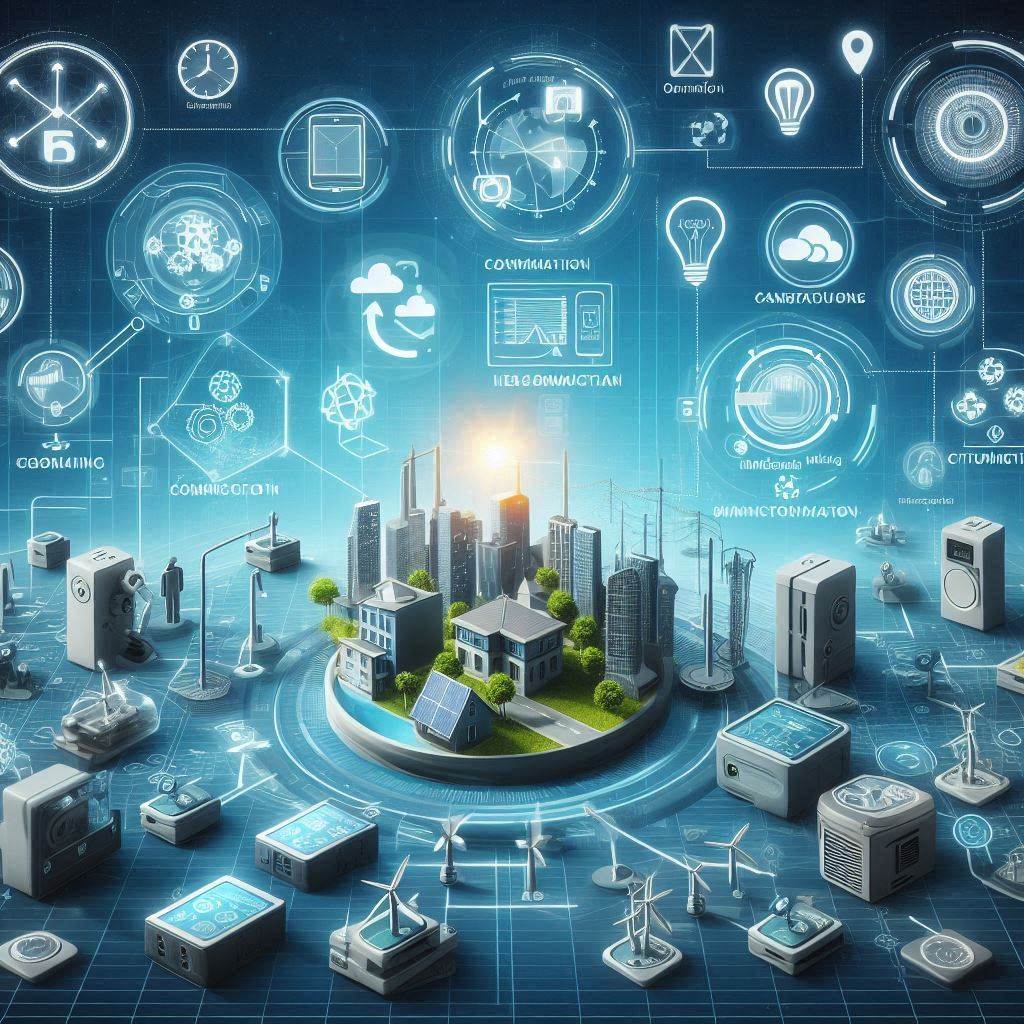
Abstract:
The project “Smart Grid Automation Using IoT” focuses on utilizing the Internet of Things (IoT) to enhance the efficiency, reliability, and security of modern power grids. The smart grid enables real-time monitoring, fault detection, and automated control, improving energy distribution and reducing energy wastage. The integration of IoT devices, including sensors and controllers, allows for efficient communication between the grid components, ensuring a reliable and sustainable power supply. The system also supports remote monitoring and control of the grid using a mobile or web application, providing valuable data for predictive maintenance and optimization.
Introduction:
As the demand for electricity continues to grow, the need for a more intelligent, reliable, and efficient energy grid has become crucial. Traditional grids often lack the capability to adapt to changing power demands or to handle the integration of renewable energy sources. The smart grid concept offers a solution by employing IoT technology to automate and optimize energy distribution and consumption. This project aims to design and implement a smart grid system using IoT for real-time monitoring, fault detection, and automated control. By doing so, the system can significantly reduce energy losses, improve reliability, and accommodate the growing adoption of renewable energy sources.
Hardware Details:
- Microcontroller (ESP32):
The ESP32 is used to control the system and handle communication between IoT devices. It processes sensor data and sends it to the cloud for real-time monitoring. - Current Sensors (ACS712):
These sensors are used to measure the current flowing through different sections of the grid. They provide the data needed for monitoring power usage and detecting faults. - Voltage Sensors (ZMPT101B):
Voltage sensors are employed to measure the voltage at various points in the grid. This data helps ensure that the grid operates within safe voltage levels. - Relay Modules:
Relays are used to control the connection and disconnection of various loads and power lines. The relays are controlled by the microcontroller, allowing the system to automate load balancing and fault management. - Wi-Fi Module (Built-in ESP32):
The ESP32’s built-in Wi-Fi module enables the system to connect to the internet, facilitating communication between the grid and the cloud for data storage and analysis. - Cloud Platform (Thingspeak or Blynk):
A cloud platform is used to store and visualize real-time data from the sensors. It also enables remote monitoring and control via a mobile app or web interface. - Power Supply:
A 5V power supply is used to power the microcontroller and other components.
Software Details:
- Arduino IDE:
The Arduino IDE is used to program the ESP32 microcontroller. It handles sensor data processing, communication with the cloud, and the control logic for grid automation. - IoT Platform (Thingspeak or Blynk):
The IoT platform is used for real-time data visualization, remote monitoring, and control. Data from sensors are sent to the platform, and control signals are sent from the platform to the relays. - Embedded C Programming:
The microcontroller code is written in Embedded C to ensure the system operates in real-time, handling sensor inputs and triggering automated responses. - Mobile App (Blynk):
A mobile app is developed using the Blynk platform to provide real-time control and monitoring of the smart grid system. Users can view power usage, fault alerts, and control loads remotely.
Coding:
#include <WiFi.h>
#include <Thingspeak.h>
const char* ssid = "your_ssid";
const char* password = "your_password";
WiFiClient client;
unsigned long myChannelNumber = 123456;
const char* myWriteAPIKey = "XYZ";
int currentSensorPin = A0;
int voltageSensorPin = A1;
int relayPin = 2;
void setup() {
Serial.begin(9600);
pinMode(relayPin, OUTPUT);
WiFi.begin(ssid, password);
while (WiFi.status() != WL_CONNECTED) {
delay(500);
Serial.println("Connecting to WiFi...");
}
Thingspeak.begin(client);
}
void loop() {
float current = analogRead(currentSensorPin) * (5.0 / 1023.0);
float voltage = analogRead(voltageSensorPin) * (5.0 / 1023.0);
Serial.println("Current: " + String(current));
Serial.println("Voltage: " + String(voltage));
if (current > 10.0 || voltage > 240.0) {
digitalWrite(relayPin, HIGH); // Turn off the load in case of a fault
} else {
digitalWrite(relayPin, LOW); // Normal operation
}
// Send data to Thingspeak
Thingspeak.setField(1, current);
Thingspeak.setField(2, voltage);
Thingspeak.writeFields(myChannelNumber, myWriteAPIKey);
delay(2000);
}
Explanation of Components:
- Microcontroller (ESP32):
The ESP32 is responsible for collecting data from sensors, processing it, and sending it to the cloud. It also controls the relays to automate the smart grid functions. - Current Sensors (ACS712):
These sensors monitor the current flowing through different grid sections and provide real-time data for load balancing and fault detection. - Voltage Sensors (ZMPT101B):
The voltage sensors monitor the grid voltage and help ensure stable operation by detecting overvoltage or undervoltage conditions. - Relay Modules:
The relays are used to control power flow in the grid. They can disconnect loads during faults or redistribute power to maintain grid stability. - Cloud Platform (Thingspeak or Blynk):
The cloud platform stores and analyzes the data collected by the sensors. It also provides a user-friendly interface for real-time monitoring and remote control.
Applications:
- Smart Energy Management:
The system can be deployed in smart grids to optimize energy distribution and improve grid reliability by monitoring energy consumption in real time. - Renewable Energy Integration:
The project can manage and balance renewable energy sources like solar and wind, ensuring efficient integration with the existing grid infrastructure. - Predictive Maintenance:
The system provides data for predictive maintenance, identifying potential faults before they occur, thus preventing major outages. - Remote Monitoring & Control:
With IoT integration, the grid can be monitored and controlled remotely through a mobile app or web interface, offering convenience and real-time control.
Advantages:
- Real-time Monitoring:
The system provides real-time data on grid performance, ensuring that any faults or inefficiencies are quickly detected and addressed. - Automation:
Smart automation of the grid reduces the need for manual intervention, improving overall reliability and efficiency. - Remote Access:
Users can monitor and control the grid remotely using the IoT platform, offering flexibility and convenience. - Scalability:
The system can be easily scaled to manage larger grids or integrated with more sensors and devices as needed. - Energy Efficiency:
The smart grid helps reduce energy wastage by optimizing energy distribution and balancing loads in real time.
Conclusion:
The Smart Grid Automation Using IoT project offers a revolutionary approach to managing modern power grids. With the integration of IoT, the system provides real-time monitoring, fault detection, and automated control, greatly improving grid efficiency and reliability. The project demonstrates how IoT technology can transform traditional power systems into smarter, more sustainable grids, capable of handling the growing demand for electricity and the integration of renewable energy. The scalable nature of the system ensures that it can be adapted to a wide range of applications, from small communities to large-scale city infrastructures.
Component Connections:
- ESP32 Microcontroller
- Current Sensor (ACS712):
- VCC: Connect to 5V pin on ESP32
- GND: Connect to GND
- Output Pin: Connect to analog pin A0 on ESP32
- Voltage Sensor (ZMPT101B):
- VCC: Connect to 5V pin on ESP32
- GND: Connect to GND
- Output Pin: Connect to analog pin A1 on ESP32
- Relay Module:
- VCC: Connect to 5V pin on ESP32
- GND: Connect to GND
- IN Pin: Connect to digital pin 2 on ESP32
- COM & NO: Connect to the load
- Wi-Fi Module (Built-in ESP32):
The Wi-Fi module is integrated into the ESP32, allowing it to connect to the cloud for data transmission and remote monitoring. - Power Supply:
The ESP32 and sensors are powered through a 5V supply, ensuring stable operation.