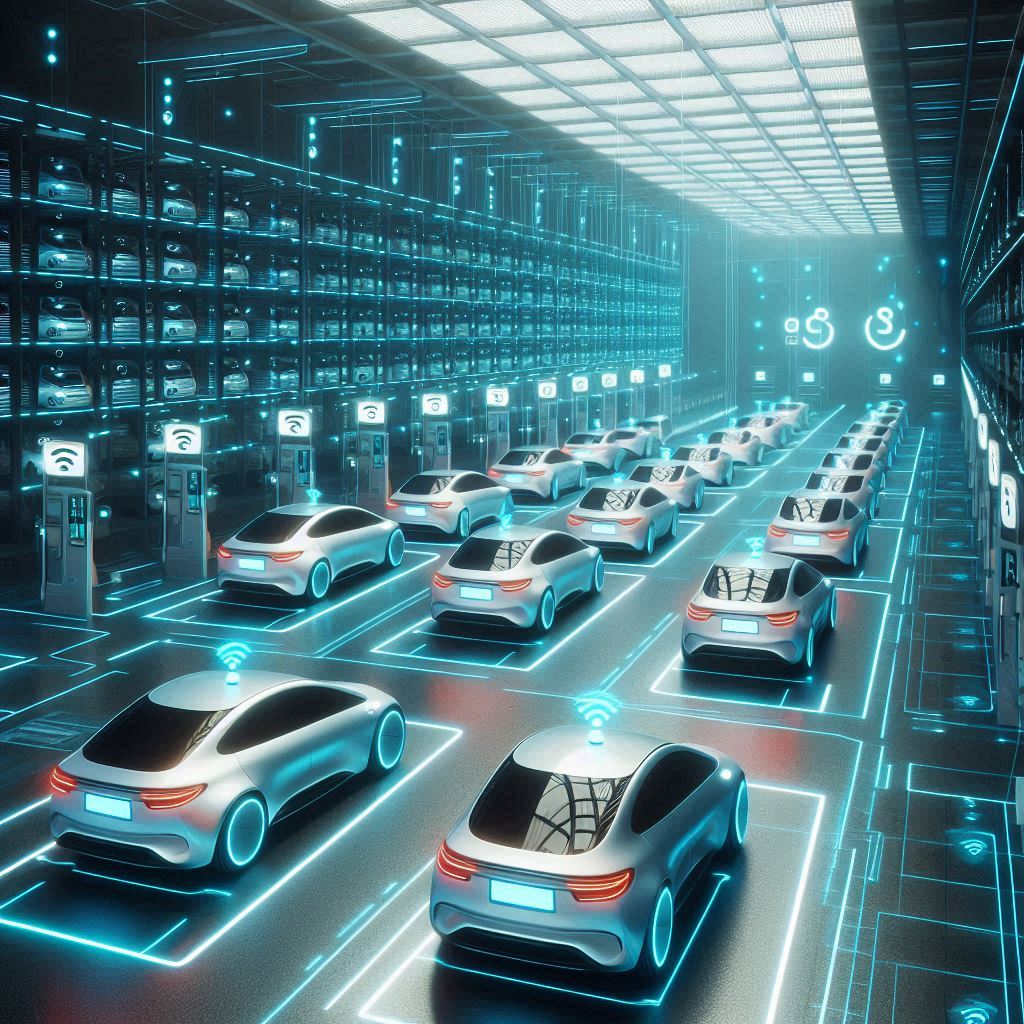
Abstract:
The GSM Autonomous Car Parking System is a cutting-edge solution designed to simplify and automate the process of vehicle parking in urban areas. The system employs a combination of GSM technology, ultrasonic sensors, and microcontrollers to autonomously navigate, detect available parking spaces, and park the car without human intervention. By using a GSM module, the system also allows remote communication with the user, providing real-time updates about the car’s location and status. The project aims to reduce the human effort and time required for parking while improving space utilization in parking lots.
Introduction:
Urban areas are facing a surge in vehicle numbers, which leads to challenges in parking management. Finding a parking space often consumes time and creates traffic congestion. This project introduces a GSM-based autonomous parking system, which utilizes a smart mechanism to park vehicles efficiently. The car parking system automatically detects the available spaces, maneuvers the vehicle into the space, and provides remote updates via GSM to the user regarding the parking status. The integration of GSM technology offers convenience and real-time communication, making this system highly adaptable to modern smart cities.
Hardware Details:
- Microcontroller (Arduino UNO or ESP32):
The microcontroller acts as the brain of the system, controlling the sensors, motors, and communication modules. It processes the sensor data and sends the necessary signals to control the car’s movement. - GSM Module (SIM800L):
The GSM module allows remote communication between the car and the user. It sends real-time messages regarding parking status, available spaces, or any issues encountered during the process. - Ultrasonic Sensors (HC-SR04):
Ultrasonic sensors are employed to detect obstacles and measure distances to ensure the car parks safely. These sensors provide feedback to the microcontroller to navigate the car into the parking spot. - Motor Driver (L298N):
The motor driver controls the movement of the DC motors, which in turn maneuver the wheels of the car. It ensures the smooth forward, backward, and turning movement of the vehicle. - DC Motors:
Two DC motors are used to drive the car. They receive signals from the motor driver to move the car forward, backward, or to steer in different directions. - Power Supply:
A 12V battery is used to power the microcontroller, sensors, and motors, ensuring the system runs autonomously without external power.
Software Details:
- Arduino IDE:
The Arduino IDE is used to write and upload the code to the microcontroller. It allows programming of the vehicle’s control logic, including sensor data interpretation, motor control, and GSM communication. - Embedded C Programming:
The microcontroller is programmed in Embedded C, enabling real-time control of the hardware components. It involves handling interrupts, sensor data, and actuating motors based on the data received. - GSM Communication Code:
AT commands are used to communicate with the GSM module. These commands are embedded within the code to send and receive SMS updates regarding parking status. - Component Connections:
- Arduino UNO Microcontroller
- Ultrasonic Sensor (HC-SR04)
- VCC: Connect to 5V pin of Arduino
- GND: Connect to GND pin of Arduino
- Trigger Pin: Connect to Arduino digital pin 9
- Echo Pin: Connect to Arduino digital pin 10
- GSM Module (SIM800L)
- VCC: Connect to 5V pin of Arduino (or external 5V power supply)
- GND: Connect to GND pin of Arduino
- RX: Connect to Arduino digital pin 7
- TX: Connect to Arduino digital pin 8
- Motor Driver (L298N)
- IN1: Connect to Arduino digital pin 3
- IN2: Connect to Arduino digital pin 4
- IN3: Connect to Arduino digital pin 5
- IN4: Connect to Arduino digital pin 6
- Motor A (left motor): Connect the left DC motor to the L298N output pins
- Motor B (right motor): Connect the right DC motor to the L298N output pins
- Power: Connect 12V battery to L298N power pins (ensure proper polarity)
- DC Motors
- The motors are controlled via the motor driver (L298N) and receive signals from the Arduino. The motor connections are handled by the L298N module, allowing the Arduino to control forward and backward movement as well as steering.
- Ultrasonic Sensor (HC-SR04)
- Power Supply (12V Battery)
Coding:
#include <SoftwareSerial.h>
#include <Ultrasonic.h>
SoftwareSerial gsm(7, 8); // RX, TX for GSM
Ultrasonic ultrasonic(9, 10); // Trigger, Echo for Ultrasonic
int motor1A = 3; // Motor 1 Pin A
int motor1B = 4; // Motor 1 Pin B
int motor2A = 5; // Motor 2 Pin A
int motor2B = 6; // Motor 2 Pin B
void setup() {
Serial.begin(9600);
gsm.begin(9600);
pinMode(motor1A, OUTPUT);
pinMode(motor1B, OUTPUT);
pinMode(motor2A, OUTPUT);
pinMode(motor2B, OUTPUT);
}
void loop() {
long distance = ultrasonic.Ranging(CM); // Get distance in CM
if (distance < 30) {
stopCar();
sendSMS("Car Parked Successfully!");
} else {
moveForward();
}
}
void moveForward() {
digitalWrite(motor1A, HIGH);
digitalWrite(motor1B, LOW);
digitalWrite(motor2A, HIGH);
digitalWrite(motor2B, LOW);
}
void stopCar() {
digitalWrite(motor1A, LOW);
digitalWrite(motor1B, LOW);
digitalWrite(motor2A, LOW);
digitalWrite(motor2B, LOW);
}
void sendSMS(String message) {
gsm.print("AT+CMGF=1\r"); // Set SMS to text mode
delay(100);
gsm.print("AT+CMGS=\"+1234567890\"\r"); // Recipient's phone number
delay(100);
gsm.print(message); // SMS content
delay(100);
gsm.write(26); // Send SMS
delay(1000);
}
Explanation of Components:
- Microcontroller:
The Arduino controls the sensors and motors. It processes data from the ultrasonic sensors to determine if the vehicle can move forward or needs to stop. - Ultrasonic Sensors:
These sensors are used to measure the distance between the vehicle and obstacles. The data is sent to the microcontroller, which then decides if the vehicle should stop or continue moving. - GSM Module:
The GSM module is used to send real-time updates to the user about the parking status. It communicates with the user’s mobile phone via SMS, using AT commands programmed into the microcontroller. - Motor Driver:
The motor driver controls the movement of the vehicle’s wheels, allowing it to turn, stop, or move forward and backward. - DC Motors:
The DC motors are responsible for physically moving the vehicle in the desired direction based on the control signals from the motor driver.
Application:
- Smart Parking Systems:
This system can be applied in public or private parking lots to automate vehicle parking and reduce the time spent searching for available spaces. - Smart City Infrastructure:
The GSM Autonomous Parking System can be integrated into smart city projects to improve parking management and space utilization. - Residential and Commercial Parking:
This system is useful in residential and commercial buildings where parking space is limited and efficient space utilization is critical.
Advantages:
- Time Efficiency:
The system automates parking, reducing the time spent by drivers searching for parking spaces. - Convenience:
With real-time updates through GSM, users are always informed about their car’s status and parking availability. - Safety:
The use of ultrasonic sensors ensures that the car is parked safely, avoiding collisions with other vehicles or obstacles. - Space Utilization:
The system maximizes the use of available parking spaces by ensuring vehicles are parked efficiently.
Conclusion:
The GSM Autonomous Car Parking System is an innovative approach to addressing the challenges of parking in urban areas. By combining GSM technology with autonomous control, it not only saves time and effort but also enhances safety and convenience. The project demonstrates how technology can be applied to real-world problems and offers a glimpse into the future of smart city infrastructure. This system has the potential to revolutionize parking management in cities, making it more efficient and user-friendly.